sandbox/acastillo/readme
Andrés Castillo 
My name is Andrés. I store in this location some of the work done in Basilisk. I mainly work with parametric instabilities, vortex instabilities and thermal convection.
Structure of this sandbox
This sandbox is divided in different parts:
Vortex Filament Method
This includes an implementation of a Vortex Filament Method (VFM) using Biot-Savart and some examples:
- Motion of a vortex ring
- Motion of 2 vortex rings
- Motion of 3 vortex rings
- Motion of an elliptical vortex ring
Motion of an elliptical vortex ring |
Motion of 2 vortex rings |
Motion of 3 vortex rings |
Output fields
This includes a series of routines to write the resulting fields into several different formats.
output_vtu() - This format is compatible with the VTK XML file format for unstructured grids File formats for VTK version 4.2 which can be read using Paraview. Here, the results are written in raw binary. The unstructured grid is required to write results from quadtrees and octrees. If used in MPI, each MPI task writes its own file, which may be linked together using a
*.pvtu
file. An example is available here.output_xmf() This routine is compatible with the XDMF Model and Format which can be read using Paraview or Visit. Data is split in two categories: Light data and Heavy data. Light data is stored using eXtensible Markup Language (XML) to describe the data model and the data format. Heavy data is composed of large datasets stored using the Hierarchical Data Format HDF5. As the name implies, data is organized following a hierarchical structure. HDF5 files can be read without any prior knowledge of the stored data. The list of software capable of reading HDF5 files includes Visit, Paraview, Matlab, and Tecplot, to name a few. The type, rank, dimension and other properties of each array are stored inside the file in the form of meta-data. Additional features include support for a large number of objects, file compression, a parallel I/O implementation through the MPI-IO or MPI POSIX drivers. Using this format requires the HDF5 library, which is usually installed in most computing centers or may be installed locally through a repository. Linking is automatic but requires the environment variables HDF5_INCDIR and HDF5_LIBDIR, which are usually set when you load the module hdf5. An example is available here.
For testing the XDMF format try:
sudo apt install libhdf5-dev hdf5-helpers hdf5-tools
export HDF5_INCDIR=/usr/include/hdf5/serial
export HDF5_LIBDIR=/usr/lib/x86_64-linux-gnu/hdf5/serial
make test_output4.tst
- For testing using MPI try:
sudo apt install libhdf5-mpi-dev hdf5-helpers hdf5-tools
export HDF5_INCDIR=/usr/include/hdf5/openmpi
export HDF5_LIBDIR=/usr/lib/x86_64-linux-gnu/hdf5/openmpi
CC='mpicc -D_MPI=4' make test_output4.tst
- After the simulation, it is possible inspect the contents of the Heavy data from the terminal using:
>> h5ls -r fields.h5
/ Group
/000000 Group
/000000/Cell Group
/000000/Cell/T Dataset {512, 1}
/000000/Cell/pid Dataset {512, 1}
/000000/Cell/u.x Dataset {512, 3}
/000000/Geometry Group
/000000/Geometry/Points Dataset {900, 3}
/000000/Topology Dataset {512, 8}
...
- output_vtkhdf() This routine is compatible with the VTKHDF File Format which can be read using Paraview or Visit. VTK HDF files start with a group called
VTKHDF
with two attributes:Version
, an array of two integers andType
, a string showing the VTK dataset type stored in the file — in our caseUnstructuredGrid
. The data type for each HDF dataset is part of the dataset and it is determined at write time. In the diagram below, showing the HDF file structure forUnstructuredGrid
, the rounded blue rectangles are HDF groups and the gray rectangles are HDF datasets. Each rectangle shows the name of the group or dataset in bold font and the attributes underneath with regular font. In our case,scalar
andvector
fields are stored asCellData
. The unstructured grid is split into partitions, with a partition for each MPI rank.
|
![]() |
|
|
An example is available here and here.
Input fields
This includes a series of routines to read existing results and use them as initial conditions for a new simulation.
- input_matrix() - This format reads a binary file written using output_matrix() and loads it into a field selected by the user. For instance, to read a square field of size L0 defined inside a regular Cartesian grid with N points, starting from (X0,Y0) stored in a file “example.bin” and load it into a scalar field,
scalar T[];
...
fprintf (stderr, "Reprising run from existing initial conditions ... \n");
fprintf (stderr, "Read from example.bin ... \n");
FILE * fp = fopen("example.bin", "r");
if (!fp) printf("Binary file not found");
input_matrix(T,fp,N,X0,Y0,L0);
fclose (fp);
...
boundary(T);
An example on how to generate some arbitrary initial condition using matlab is available here
- initial_condition_2Dto3D() - This function reads 2D simulation results from a binary file in a format compatible with the gnuplot binary matrix format in double precision, see auxiliar_input.h. The 2D results are then used to initialize a 3D simulation, which may be useful to reduce computational cost by avoiding long transients, or to focus on the development of 3D instabilities from a 2D base state.
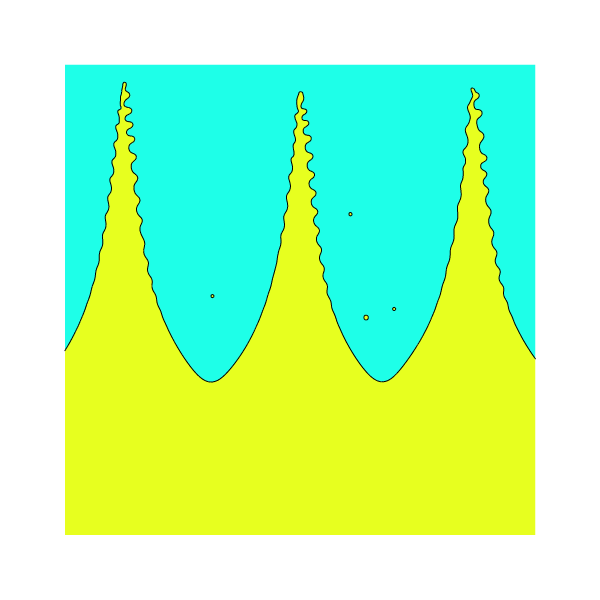
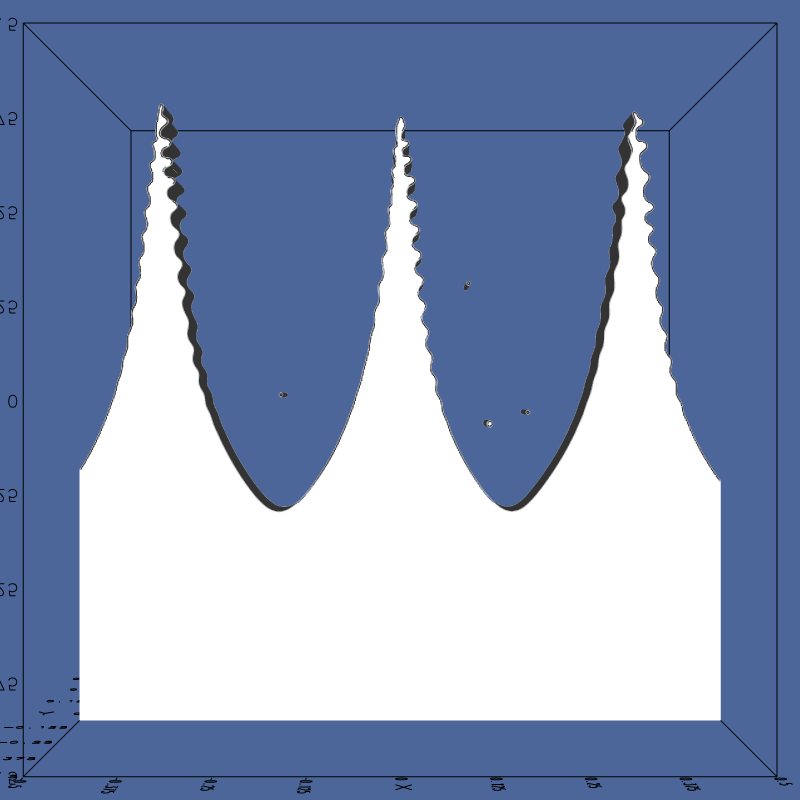
- initial_conditions_dimonte_fft2() This can be used to initialize an interface using an annular spectrum as in Dimonte et al. (2004).
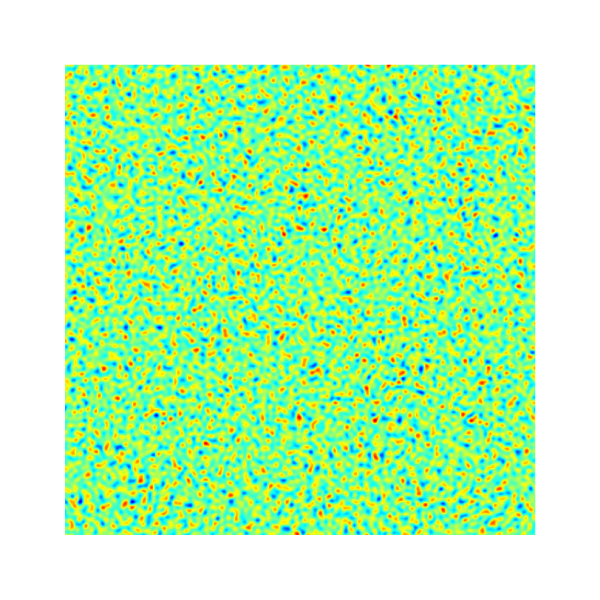
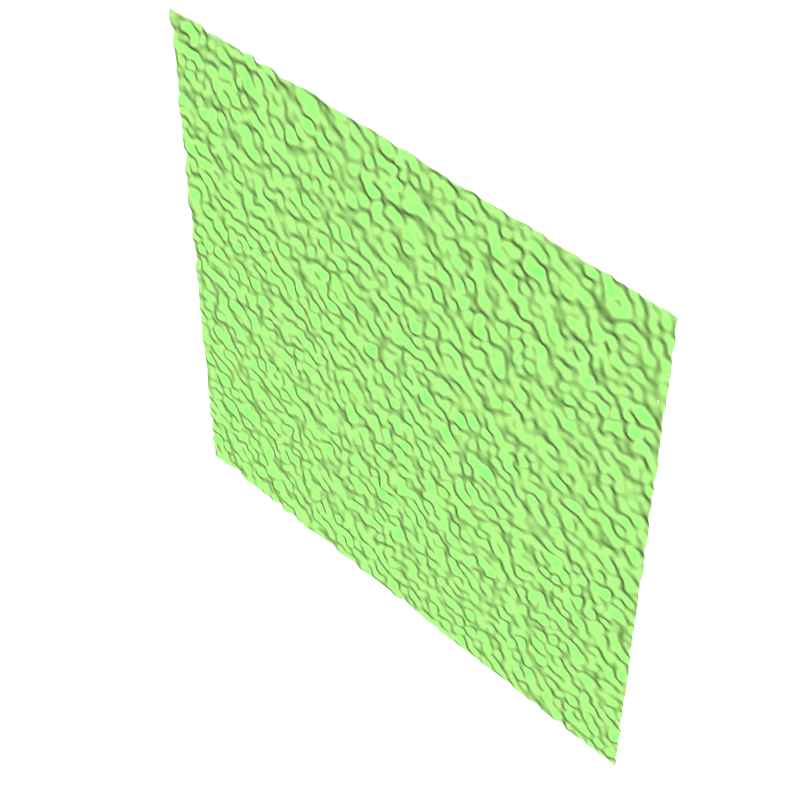
isvof=0
(left) and isvof=1
(right).
Articles Submitted or in Preparation
Parametric instabilities
[Castillo2025] |
Andrés Castillo-Castellanos, Benoît-Joseph Gréa, Antoine Briard, and Louis Gostiaux. Mixing induced by faraday surface waves. In preparation for Journal of Fluid Mechanics, 2025. |
[Grea2025] |
Benoît-Joseph Gréa, Andrés Castillo-Castellanos, Antoine Briard, Alexis Banvillet, Nicolas Lion, Catherine Canac, Kevin Dagrau, and Pauline Duhalde. Frozen waves in the inertial regime. Submitted to Journal of Fluid Mechanics, 2025. |
Articles in Publication
Vortex flows
[abraham2022] |
Aliza Abraham, Andrés Castillo-Castellanos, and Thomas Leweke. Simplified model for helical vortex dynamics in the wake of an asymmetric rotor. FLOW, applications of fluid mechanics, 3:E5, 2023. [ DOI | http | .pdf ] |
[castillo2022] |
Andrés Castillo-Castellanos and S Le Dizès. Closely spaced co-rotating helical vortices: long-wave instability. Journal of Fluid Mechanics, 946, August 2022. [ DOI | http | .pdf ] |
[castillo2021] |
A Castillo-Castellanos, Stéphane Le Dizès, and E Durán Venegas. Closely spaced co-rotating helical vortices: General solutions. Physical Review Fluids, 6(11):114701, 2021. [ DOI | http | .pdf ] |
Thermal convection
[castillo2019] |
Andrés Castillo-Castellanos, Anne Sergent, Bérengère Podvin, and Maurice Rossi. Cessation and reversals of large-scale structures in square Rayleigh-Bénard cells. Journal of Fluid Mechanics, 877:922–954, October 2019. [ DOI | http | .pdf ] |
[castillo2017] |
Andrés Alonso Castillo-Castellanos. Turbulent convection in Rayleigh-Bénard cells with modified boundary conditions. Theses, Université Pierre et Marie Curie - Paris VI, September 2017. [ http | .pdf ] |
[castillo2016] |
Andrés Castillo-Castellanos, Anne Sergent, and Maurice Rossi. Reversal cycle in square Rayleigh-Bénard cells in turbulent regime. Journal of Fluid Mechanics, 808:614–640, 2016. [ DOI | http | .pdf ] |
References
[dimonte2004] |
Guy Dimonte, D. L. Youngs, A. Dimits, S. Weber, M. Marinak, S. Wunsch, C. Garasi, A. Robinson, M. J. Andrews, P. Ramaprabhu, A. C. Calder, B. Fryxell, J. Biello, L. Dursi, P. MacNeice, K. Olson, P. Ricker, R. Rosner, F. Timmes, H. Tufo, Y.-N. Young, and M. Zingale. A comparative study of the turbulent rayleigh–taylor instability using high-resolution three-dimensional numerical simulations: The alpha-group collaboration. Physics of Fluids, 16(5):1668–1693, 05 2004. [ DOI ] |